I said:
private var pos:Vector3;
function Move(dir:Vector3)
{
pos = dir;
}
function FixedUpdate()
{
var hit:RaycastHit;
if(Physics.Raycast(transform.position+Vector3(0,5,0), Vector3(0,-1,0), hit, Mathf.Infinity, 1<<8))
{
rigidbody.position.y = hit.point.y + (collider.bounds.size.y/2);
}
if(pos.magnitude != 0)
{
var dir:Vector3 = pos*GetComponent(Unit).speed*Time.deltaTime;
if(Physics.Raycast(transform.position, pos, hit, collider.bounds.size.z/1.5, 1<<9))
{
dir *= -1;
GetComponent(Unit).currentWaypoint = GetComponent(Unit).path.vectorPath.Length;
}
if(dir.magnitude < .375)
{
dir *= 0;
}
rigidbody.MovePosition(rigidbody.position+dir);
pos *= 0;
}
}
==================================================
private var seeker:Seeker;
//The calculated path
var path:Pathfinding.Path;
//The AI's speed per second
var speed: float = 20;
var range: float = 100;
//The max distance from the AI to a waypoint before it can continue to the next waypoint
private var previousPosition:Vector3;
var nextWaypointDistance: float = 3; // 2
private var commandPoint:Vector3;
//The waypoint we are currently moving towards
var currentWaypoint: int = 0;
private var squadCenter:Vector3;
private var flocking = false;
var priority = 0;
function Start()
{
seeker = GetComponent(Seeker);
}
// New Function
function relativeCenter(center:Vector3)
{
squadCenter = center;
}
// New Function
function flock(shouldFlock:boolean)
{
flocking = shouldFlock;
}
// Updated Function
function Command(hit:RaycastHit)
{
if(hit.collider.GetComponent(Selectable) != null)
{
if(GetComponent(Selectable).team == hit.collider.GetComponent(Selectable).team)
{
if(hit.collider.GetComponent(Building) != null)
{
}
else
{
if(hit.collider.GetComponent(Unit) != null)
{
}
if(hit.collider.GetComponent(Building) != null)
{
}
}
}
else
{
Move(hit.point);
}
}
}
//Updated function
function Move(point:Vector3)
{
commandPoint = point;
if(!flocking)
{
var offset = transform.position - squadCenter;
point += offset;
}
seeker.StartPath(transform.position, point, MyCompleteFunction);
}
//Updated function
function MyCompleteFunction(p:Pathfinding.Path)
{
if (!p.error)
{
var end = p.vectorPath[p.vectorPath.Length-1];
end.y = commandPoint.y;
if(Physics.Linecast(commandPoint, end, 1<<9))
{
seeker.StartPath(transform.position, commandPoint, MyCompleteFunction);
}
path = p;
currentWaypoint = 0;
}
}
//New function
function OnCollisionStay(col:Collision)
{
if(col.transform.GetComponent(Unit) != null)
{
if(GetComponent(Selectable).team == col.transform.GetComponent(Selectable).team)
{
var pos:Vector3;
if(priority == 0 && col.transform.GetComponent(Unit).priority == 0)
{
pos = Vector3.Normalize(col.transform.position-transform.position);
col.transform.GetComponent(Mover).Move(pos);
}
if(priority > col.transform.GetComponent(Unit).priority)
{
pos = Vector3.Normalize(col.transform.position-transform.position);
col.transform.GetComponent(Mover).Move(pos);
}
}
}
if(col.transform.GetComponent(Building) != null)
{
}
}
//Updated function
function Update()
{
if(path == null)
{
//We have no path to move to
return;
}
if(currentWaypoint >= path.vectorPath.Length)
{
//End of path
flocking = false;
priority = 0;
return;
}
//Direction to the next waypoint
priority = 1;
var dir = Vector3.Normalize(path.vectorPath[currentWaypoint]-transform.position);
transform.LookAt(Vector3(path.vectorPath[currentWaypoint].x, transform.position.y, path.vectorPath[currentWaypoint].z));
GetComponent(Mover).Move(dir);
if(Vector2.Distance(Vector2(transform.position.x, transform.position.z), Vector2(path.vectorPath[currentWaypoint].x, path.vectorPath[currentWaypoint].z)) < nextWaypointDistance)
{
currentWaypoint++;
return;
}
}
Unity said:

I said:
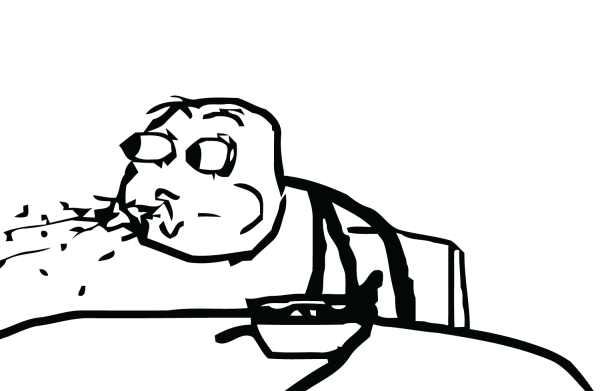